The Definitive Command Cheat Sheet for Rails Beginners. March 7th 2020 1,683 reads @melbeavsmelbeavs When I was a newbie in Rails, the first couple of weeks I. Rails uses the cookie-based approach to session data. Users of Rails applications must have cookies enabled in their browsers. Any key/value pairs you store into this hash during the processing of a request willbe available during subsequent request from the same browser. Rails stores session information in a file on the server. Be careful if you.
Rails database migrations cheat-sheet; Rails query cache; Redirect back with Rails; Reusing Rails scopes in the OR operator; Running tests with Minitest; self vs extend self vs modulefunction; Simple HTTP server with Ruby; Super and dynamically created methods in Ruby; Turning string into predicate in Ruby; Using auto-loaded constants in Rails. In Rails, callbacks are hooks provided by Active Record that allow methods to run before or after a create, update, or destroy action occurs to an object. Since it can be hard to remember all of them and what they do, here is a quick reference for all current Rails 5 Active Record callbacks.
Ruby is an easy language to learn, but it's often necessary to look up something we've forgotten. A combination of Google plus any Ruby books we have on our shelves can help, but sometimes it's handy to refer to a simpler set of notes - such as a 'cheat sheet.' This post attempts to cover the most interesting ones.

The idea for this initial list came from Scott Klarr's own list. Scott has been quite prolific lately in putting together lists of cheat sheets. Some of his lists are: Apache cheat sheets, MySQL cheat sheets, PHP cheat sheets, and JavaScript / AJAX cheat sheets.
On with the Ruby cheat sheets:
'Essential Ruby' RefCard (PDF)
Essential Ruby is a combination of a cheat sheet and a tutorial. It's six pages long, but features a mini Ruby introduction and tutorial, as well as the myriad of tables you'd expect from a cheat sheet. It's like a whole Ruby beginner's reference and tutorial in a single PDF. The only 'catch' is that you need to be a user of DZone (the 'Digg for Developers') or JavaLobby to get it, but there's a very simple signup form you can fill in if you're not.
Ruby Cheatsheet (PDF and PNG)
This cheat sheet (homepage) covers types, exceptions, expressions, variable types, operators and precedence, constants, regular expressions, predefined / special variables, arguments accepted by the Ruby interpreter, reserved words, and a large collection of Object, String, Kernel, Array, Hash, Test::Unit, File, Dir and DateTime methods. Extremely suitable for printing and/or wall chart use.
Ruby QuickRef (HTML)
I often use this one myself! A ridiculously comprehensive 'cheat sheet' created by Ryan Davis covering syntax rules, reserved words, regular expression terminology, class and method definitions, predefined variables and constants, control and logic expressions and formations, exceptions, the standard library, and a whole ton of useful stuff.
Ruby Reference (PDF)
More of an extremely brief reference than a truly comprehensive cheat sheet, but still useful, especially for beginners. 5 pages long, so not suitable for printing (Also available to view on the Web at Scribd.)
Cheat: Ruby Cheat Sheets on your Terminal! (Application)
Not a cheat sheet, but an application (gem install cheat) that provides cheat sheet type reference material on your terminal (from 228 source cheat sheets!) Developed by the great guys over at Err Free.
A Beginner's Notes (HTML)
A set of notes made by a beginning Ruby developer as he works his way through a Ruby manual.
Ruby Command Line Parameters Cheat Sheet (HTML)
A simplified list of the command line options offered by the Ruby interpreter.
Strftime Parameters (HTML)
A handy guide to the formatting parameters accepted by strftime. I commonly have to look these up myself!
- Name
- Austin Miller
- @armiiller
We’ve been doing some Ruby on Rails development lately, in preparation for PagerTree 4, and we wanted to put together a Ruby on Rails Cheat sheet. This is a quick reference guide to common ruby on rails commands and usage.
Visual studio code ipad. Table of Contents:
- Ruby Syntax
- Rails Framework
- Useful Things
Ruby Syntax
Hashes
Hashes were one of the most confusing things to me when first starting ruby (not because they are a new concept, but because I found the syntax very hard to read). In newer versions, syntax is very similar to JSON notification. Just know there are two versions of syntax, an older and newer one.
Also, you can have symbols as keys for hashes, and they do not lookup the same values as strings.
Safe Navigation Operator
Instead of checking for nil or undefined values, you can use the safe navigation operator. There’s a nice article here that goes into more depth of explanation.
ERB
Evaluation and Output
Evaluation can be done with the <% %>
syntax and output can be achieved with the <%= %>
syntax.
Partials
You can render partials like so:
Extract the macOS Catalina Virtual Image. When you first get the Virtual Image it will be in what is. VirtualBox Boot Image File: (Google Drive – Direct Link) The file is zipped and compressed into a single working file which you can use to install macOS Catalina on VirtualBox easily by booting into, erasing the drive and clicking install to get it installed. It is completely without password. Download macOS Catalina ISO. You’ve to download macOS Catalina ISO from the link provided. Virtualbox macos catalina image. VirtualBox 6.1.2 will not run Catalina 10.15.2 and later guests. Virtualbox 6.1.0 added support for booting APFS.Unfortunately there is a problem if you do not have 10.15.1 version of Install macOS Catalina.app. There is a bug in VirtualBox which is exposed by 10.15.2 and later versions of boot.efi.See this VirtualBox forum thread Catalina 10.15.2 does not start. Download macOS Catalina ISO. You have to get macOS Catalina ISO in the link supplied.
Rails commands
Rails Activerecord Cheat Sheet
Common rails commands. (Note: “rails g” stands for “rails generate”)
Command | Description |
---|---|
rails g model user name:string age:integer account:references | Generates model and migration files |
rails g scaffold user name:string age:integer account:references | Generates controller, model, migration, view, and test files. Also modifies config/routes.rb |
rails g scaffold_controller user | Generates controller and view files. Useful if you already have the model |
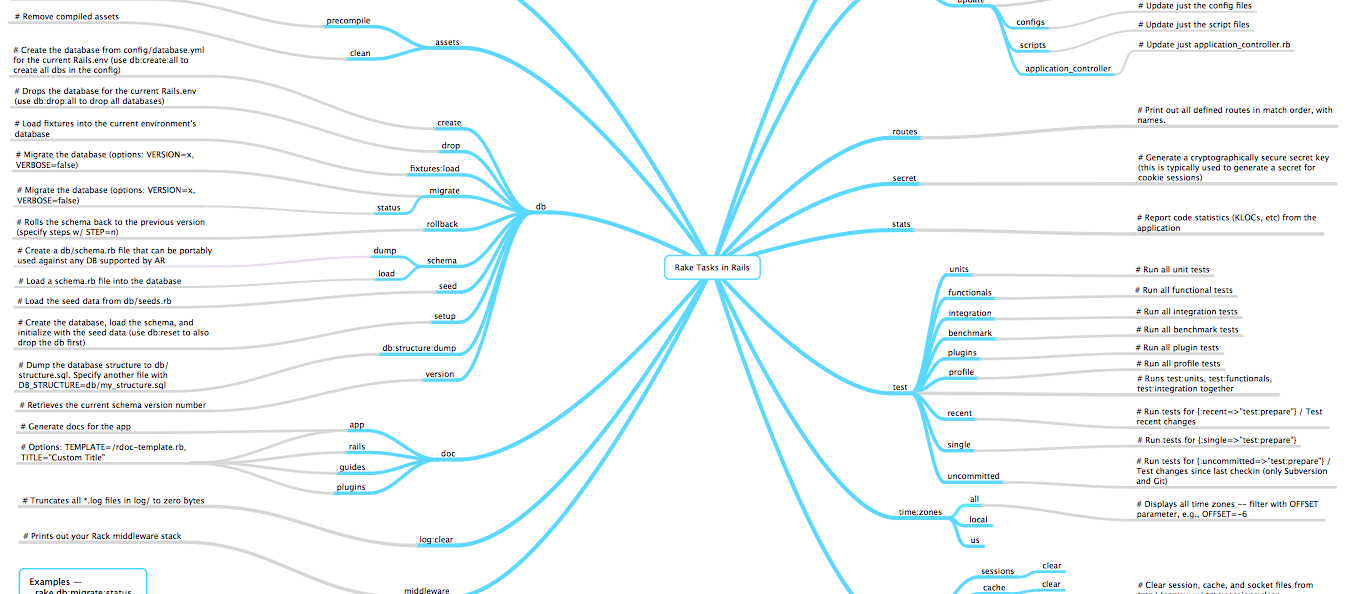
Rake Commands
Common rake commands for database management and finding routes.
Command | Description |
---|---|
rake routes | View all routes in application (pair with grep command for some nifty searching) |
rake db:seed | Seed the database using the db/seeds.rb |
rake db:migrate | Run any pending migrations |
rake db:rollback | Rollback a database migration (add STEP=2 to remove multiple migrations) |
rake db:drop db:create db:migrate | Destroy the database, re-created it, and run migrations (useful for development) |
Gitlab Rails Cheat Sheet

Ruby Cheat Sheet Pdf
Rails Framework
Migration Data Types
Migration data types. Here is the source and a stack overflow question I commonly reference.
- :boolean
- :date
- :datetime
- :decimal
- :float
- :integer
- :primary_key
- :references
- :string
- :text
- :time
- :timestamp
Controller Filters
Filters are methods that are run “before”, “after” or “around” a controller action. See full action controller filters documentation for details.
Before filters are registered via the before_action
and can halt the request cycle.
Models Callbacks
Gusto has a really nice article one best practices for model callbacks.
This table references the ruby on rails documentation for active record callbacks. Check out the full documentation for other special callbacks like after_touch
.
New Record | Updating Record | Destroying Record |
---|---|---|
save | save | destroy |
save! | save! | destroy! |
create | update_attribute | |
create! | update | |
update! | ||
before_validation | before_validation | |
after_validation | after_validation | |
before_save | before_save | |
around_save | around_save | |
before_create | before_update | before_destroy |
around_create | around_update | around_destroy |
after_create | after_update | after_destroy |
after_save | after_save | |
after_commit / after_rollback | after_commit / after_rollback | after_commit / after_rollback |
Model Queries
A couple of basic (and most commonly used) queries are below. You can find the full documentation here.
Command Example | Description |
---|---|
Model.find(10) | Find model by id |
Model.find_by({ name: 'Austin' }) | Find models where conditions |
Model.where('name = ?', params[:name]) | Find models where condition |
Model.where.not('name = ?', params[:name]) | Find models where condition not true |
Model.first | Get the first model in the collection (ordered by primary key) |
Model.last | Get the lst model in the collection (ordered by primary key) |
Model.order(:created_at) | Order your results or query |
Model.select(:id, :name) | Select only specific fields |
Model.limit(10).offset(20) | Limit and offset (great for pagination) |
Fastest Check For Existence
Rails Migration Cheat Sheet
Additionally, you are likely to want to check for an existence of a condition many times. There are many ways to do this, namely present?, any?, empty?, and exists? but the exists?
method will be the fastest. This semaphore article explains nicely why exists?
is the fastest method for checking if one of a query exists.
Application Configuration
Application configuration should be located in config/application.rb
with specific environment configurations in config/environments/
. Don’t put sensitive data in your configuration files, that’s what the secrets are for. You can access configurations in your application code with the following:
Application Secrets
Application secrets are just that, secret (think API keys). You can edit the secrets file using the following commands rails credentials:edit --environment=env_name
. This will create files in the config/credentials/
folder. You’ll get two files:
environment.yml.enc
- This is your secrets encrypted - This can be put this into gitenvironment.key
- This contains the key that encrypts the file - DO NOT put this into git.
Additionally, when deploying, the key inside the environment.key
file will need to be placed into the RAILS_MASTER_KEY
environment variable. You can then access secrets in your rails code like so:
Useful Things
A short list of gems, frameworks and education materials that I have found useful in my Rails journey.
Gems
- Acts as Tenant - Easy multi-tenancy for rails database models.
- Administrate - Rails engine for flexible admin dashboard.
- Devise - Flexible authentication system.
- Devise Masquerade - Provides “Login As” another user functionality for Devise.
- Faker - Generate fake data like names, addresses, and phone numbers. Great for test data.
- Hash Id - Expose a hashid instead of primary id to your users.
- Local Time - Display friendly client side local time.
- Lockbox - Encryption for database fields (model attributes).
- Pagy - Gold standard pagination gem.
- Rack Attack - Rack middleware (before Rails) for blocking & throttling.
- Recaptcha - A rails Google Recaptcha plugin - You’ll want this one especially for public facing forms to stop bot crawlers.
- StimulusJS - A tiny framework for sprinkles of Javascript for your front end.
- Sequenced - Generate scoped ids (ex: per tenant ids for models, aka friendly id).
- Sidekiq - Redis backed background processing for jobs.
- Sidekiq Cron - A scheduler for Sidekiq (think running weekly email reports).
- Turbolinks - Makes web app feels faster (like single page application).
Frameworks
- Jumpstart Rails - A SaaS Framework already supporting login, payment (Stripe, Paypal, and Braintree) and multi-tenant setup.
- tailwindcss - A utility first CSS framework. Seems a little verbose at first, but you’ll really learn to love it. Just by reading the code, you’ll know exactly what the screen will look like.
Education
- Go Rails - Ruby on Rails tutorials, guids, and screencasts.
I hope you find some value in this cheat sheet. There’s probably a lot I missed on here, so if you have something to add you can reach out to me on twitter and I will update the article with your suggestion.

Comments are closed.